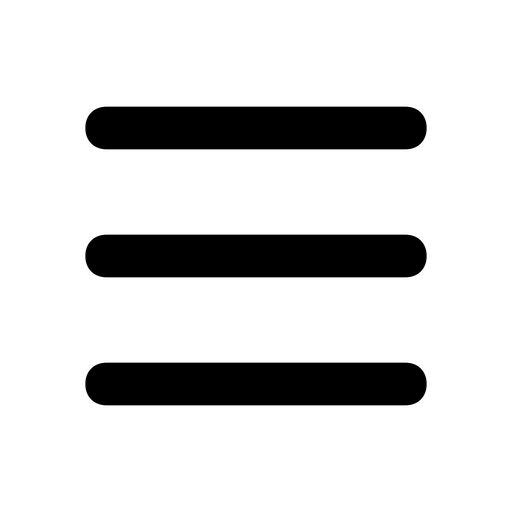
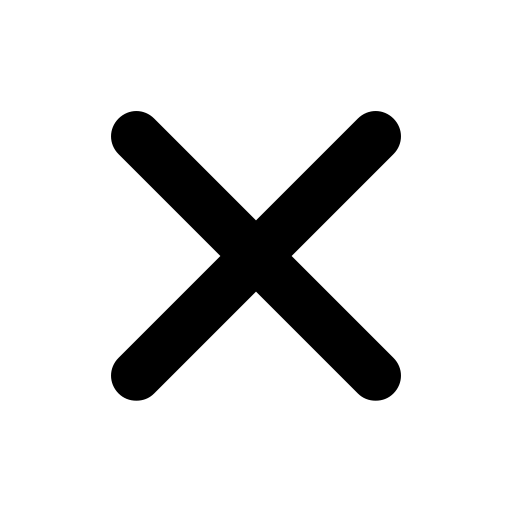
Blogs by Gulshan - the chuparushtam
Learn JavaScript - in fun way!
JavaScript is a popular programming language used for creating dynamic web content and interactive user interfaces. It is one of the core technologies of the web, along with HTML and CSS. JavaScript is a versatile language that can be used for everything from simple client-side form validation to complex full-stack web applications. In this blog, we will discuss the key features of JavaScript and explore a real-world example that demonstrates how it can be used to create a dynamic and engaging user interface.
Key Features of JavaScript
- JavaScript allows web developers to create interactive user interfaces that respond to user actions in real-time. This is achieved by using event-driven programming, which allows the program to respond to user actions such as clicks, keystrokes, and mouse movements.
- scripting: JavaScript is primarily used as a client-side scripting language, meaning that it runs in the user's web browser rather than on the web server. This enables web developers to create rich, dynamic user interfaces that are highly responsive and engaging.
- compatibility: JavaScript is supported by all modern web browsers, including Chrome, Firefox, Safari, and Edge. This ensures that web applications built using JavaScript will work on any device with a modern web browser, regardless of the operating system or hardware.
- Extensibility: JavaScript is highly extensible and can be extended through the use of third-party libraries and frameworks. This allows web developers to leverage existing code and tools to accelerate development and improve the quality of their code.
Real-World Example: Interactive To-Do List
To demonstrate the power of JavaScript, we will build an interactive to-do list application that allows users to add, edit, and delete tasks in real-time. This example will showcase some of the key features of JavaScript, including event-driven programming, client-side scripting, and cross-platform compatibility.
Step 1: Set Up the HTML Structure
To begin, we will set up the HTML structure of our to-do list application. We will create a simple form that allows users to enter a task, and a table that displays the list of tasks.
<!DOCTYPE html> <html> <head> <title>JavaScript To-Do List</title> <style> /* Add some basic styles to the table */ table { border-collapse: collapse; margin-top: 20px; } td { padding: 5px; border: 1px solid #ccc; } </style> </head> <body> <h1>JavaScript To-Do List</h1> <form> <label for="task">Task:</label> <input type="text" id="task" name="task"> <button type="submit">Add Task</button> </form> <table> <thead> <tr> <th>Task</th> <th>Action</th> </tr> </thead> <tbody id="task-list"></tbody> </table> </body> </html>
Step 2: Add JavaScript to Handle Form Submission
Next, we will add some JavaScript to handle the form submission and add the task to the to-do list. We will use event-driven programming to listen for the form submission event and prevent the default behavior of the form. We will then get the value of the task input field and add it to the table.
// Get a reference to the form and the task list const form = document.querySelector('form'); const taskList = document.querySelector('#task-list'); // Listen for the form submission event form.addEventListener('submit', (event) => { // Prevent the default behavior of the form event.preventDefault(); // Get the value of the task input field const taskInput = document.querySelector('#task'); const task = taskInput.value; // Create a new row in the task list table const row = document.createElement('tr'); const taskCell = document.createElement('td'); const actionCell = document.createElement('td'); const removeButton = document.createElement('button'); const removeButtonText = document.createTextNode('Remove'); // Set the task cell text and append it to the row taskCell.textContent = task; row.appendChild(taskCell); // Add the remove button to the action cell removeButton.appendChild(removeButtonText); actionCell.appendChild(removeButton); row.appendChild(actionCell); // Add the new row to the task list taskList.appendChild(row); // Clear the task input field taskInput.value = ''; });